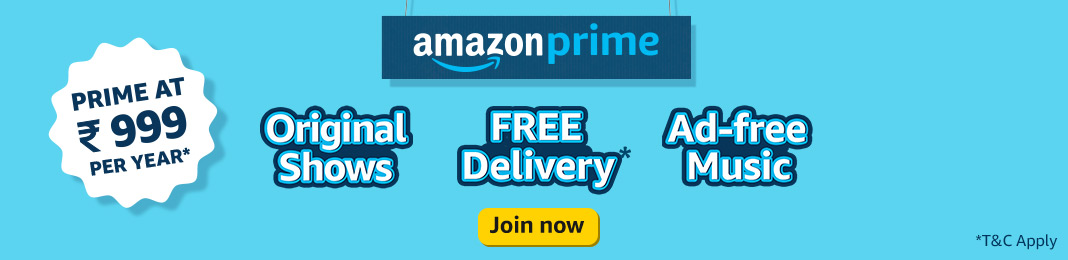
Largest of Two Numbers
Problem Statement
1Problem Description : Find the largest of two numbers.23Example 1 :4Input : a = 3 and b = 75Output : 76Explanation : 7 is the largest number.78Example 2 :9Input : a = 12 and b = 710Output : 1211Explanation : 12 is the largest number.
Algorithm
1Input : Two numbers a and b2Step 1: Start3Step 2: Read number a4Step 3: Read number b5Step 4: if a > b , print a (Compare a and b using greater than operator)6Step 5: else print b7Step 6: Stop8Output : Largest number among a and b
Video Explanation
Flow Chart
Code Implementation
- Java
- C
1import java.util.Scanner;23public class LargestOfTwoNumbers {45 public static void main(String[] args) {67 int a, b;8 Scanner sc = new Scanner(System.in);910 System.out.print("Enter a: ");11 a = sc.nextInt();12 System.out.print("Enter b: ");13 b = sc.nextInt();1415 if (a > b) {16 System.out.printf("Largest Number is : %d \n", a);17 } else {18 System.out.printf("Largest Number is : %d \n", b);19 }20 }21}