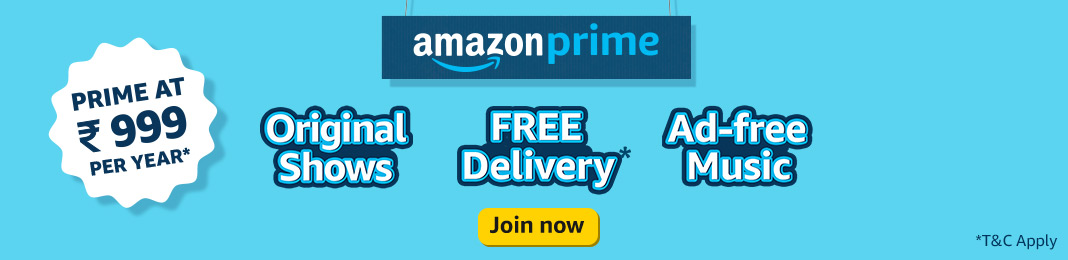
Sum of Three Numbers
Problem Statement
1Problem Description : Write Algorithm, Flowchart and Program to Add Three Integers23Example 1 :4 Input : num1 = 3, num2 = 7 and num3 = 55 Output : 156 Explanation : sum of 3, 7 and 5 results to 1578Example 2 :9 Input : num1 = 12, num2 = 7 and num3 = 110 Output : 2011 Explanation : sum of 12, 7 and 1 results to 20
Algorithm
1Input : Three numbers num1, num2 and num323Step 1: Start45Step 2: Declare sum to 06 (This is optional step, during step57 we can add declaration and assign directly as well)89Step 3: Read number num11011Step 4: Read number num21213Step 5: Read number num31415Step 5: Add num1, num2 and num3 and assign result to variable sum1617Step 6: Print sum1819Step 7: Stop2021Output: Sum of num1, num2 and num3
Flowchart
Code Implementation
- Java
- C
1import java.util.Scanner;23public class SumThreeNumbers {45 public static void main(String[] args) {6 Scanner sc = new Scanner(System.in);78 System.out.print("Enter num1: \n");9 int num1 = sc.nextInt();1011 System.out.print("Enter num2: \n");12 int num2 = sc.nextInt();1314 System.out.print("Enter num3: \n");15 int num3 = sc.nextInt();1617 int sum = num1 + num2 + num3 ;18 System.out.printf("sum of %d, %d, %d = %d \n", num1, num2, num3, sum);19 }20}
Output 1 :
1Enter num1: 122Enter num2: 73Enter num3: 1412 + 7 + 1 = 20
Output 2 :
1Enter num1: 32Enter num2: 73Enter num3: 543 + 7 + 5 = 15