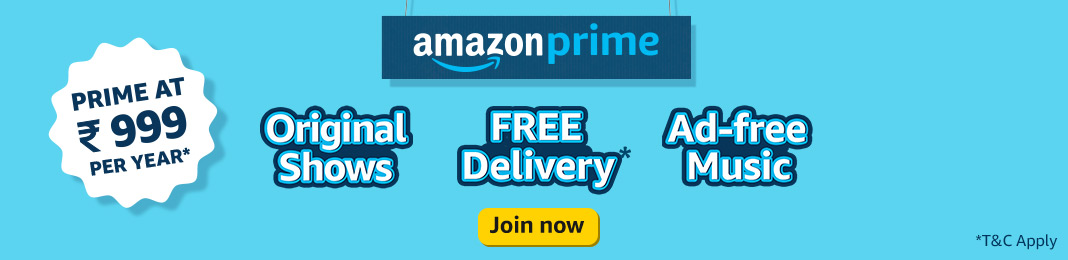
Sum of n Input Numbers
Problem Statement
1Problem Description :2Write Algorithm, Flowchart and Program to find sum of n input numbers34Example 1 :5 Input : n = 4 and the numbers are 5, 3, 7, 46 Output : 197 Explanation : sum of 5, 3, 7 and 4 is 1989Example 2 :10 Input : n = 3 and the numbers are 5, 3, 711 Output : 1512 Explanation : sum of 5, 3 and 7 results to 15
Algorithm
1Input : n and n input numbers2Step 1: Start3Step 2: Read number n4Step 3: Declare sum to 0 and i to 1 (Here i is a counter variable which is used to input n numbers)5Step 4: Repeat steps 5 to 7 until i<=n6Step 5: Read number num7Step 6: update sum as sum = sum + num8Step 7: increment i9Step 8: Print sum10Output: sum
Video Explanation
Flowchart
Code Implementation
- Java
- C
1import java.util.Scanner;23public class SumOfNInputNumbers {45 public static void main(String[] args) {6 Scanner sc = new Scanner(System.in);78 // Read n9 System.out.print("Enter n : ");10 int n = sc.nextInt();1112 // Declare sum and counter variable i13 int sum = 0;14 int i;1516 // loop to input n numbers17 // loop continues until i<=n18 for (i = 1; i <= n; i++) {19 // Read number20 System.out.printf("Enter number%d: ", i);21 int number = sc.nextInt();2223 // update sum24 sum = sum + number;25 }26 // Print sum27 System.out.printf("Sum is %d \n", sum);28 }29}