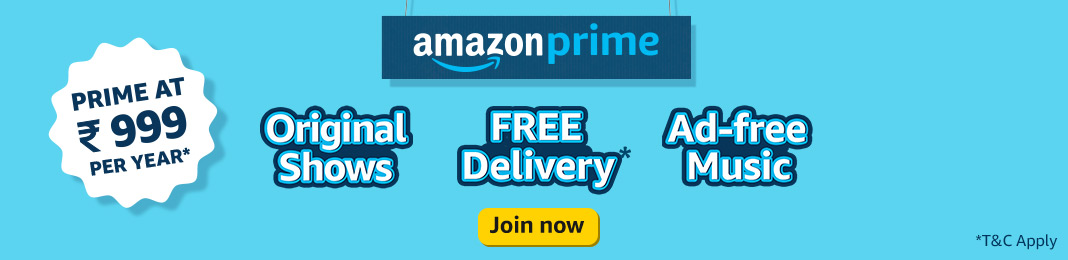
Sum of Two Numbers with Sum > 20
Problem Statement
1Problem Description :2Write algorithm and a flowchart that will compute the sum of two numbers. if the sum is below or equal to twenty, two numbers will be entered again. if the sum is above 20, it will display the sum.
Algorithm
1Input : Two numbers num1 and num22Step 1: Start3Step 2: Declare sum to 0 (This is optional step, during step5 we can add declaration and assign directly as well)4Step 3: Read number num15Step 4: Read number num26Step 5: Add num1 and num2 and assign result to variable sum7Step 6: If sum is greater than 20 Goto Step 7, else Goto Step 38Step 7: Print sum9Step 8: Stop10Output: Sum of num1 and num2
Flowchart
Code Implementation
- Java
- C
1import java.util.Scanner;23public class SumOfTwoNumbersWithSumGreaterThan20 {45 public static void main(String[] args) {6 // Initialize scanner to which is used to read inputs7 Scanner sc = new Scanner(System.in);89 while (true) {10 // Read number1 and number 211 System.out.print("Enter num1: ");12 int num1 = sc.nextInt();13 System.out.print("Enter num2: ");14 int num2 = sc.nextInt();1516 // calculate sum17 int sum = num1 + num2;1819 // if sum is greater than 20 print it20 // and break the loop21 if (sum > 20){22 System.out.printf("Sum is %d \n", sum);23 break;24 }25 // continue for next input26 System.out.printf("sum: %d is less than 20, Try again\n", sum);27 }28 }29}
Output 1 :
1Enter number1: 12Enter number2: 23sum: 3 is less than 20, Try again4Enter number1: 15Enter number2: 4361 + 43 = 44
Output 2 :
1Enter number1: 212Enter number2: 12321 + 12 = 33