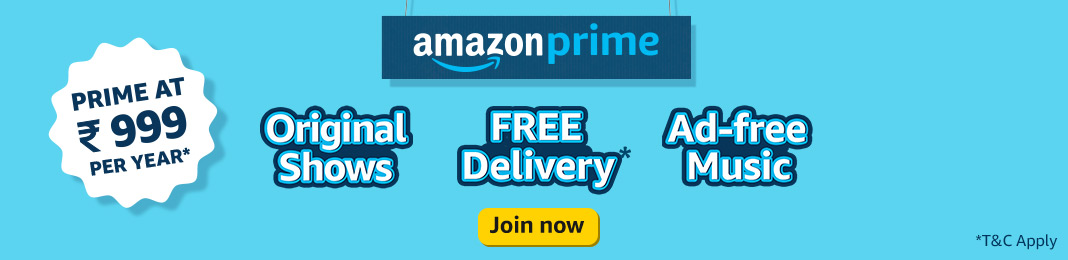
Java program to print Odd Even Numbers Using Two Threads and Semaphore
In this tutorial, we will learn to print odd and even numbers sequentially using two threads. Like odd thread should print odd numbers and even thread should print even numbers in sequential order. For this two threads should communicate with each other which is called inter-thread communication
Code Implementation
1import java.util.concurrent.Semaphore;23public class OddEvenPrinterUsingSemaphore {45 Semaphore evenSemaphore = new Semaphore(0);6 Semaphore oddSemaphore = new Semaphore(1);78 public static void main(String[] args) {9 int max = 20;10 OddEvenPrinterUsingSemaphore obj = new OddEvenPrinterUsingSemaphore();11 Thread t1 = new Thread(() -> {12 for (int i = 1; i <= max; i = i + 2) {13 obj.printOdd(i);14 }15 }, "T1");16 Thread t2 = new Thread(() -> {17 for (int i = 2; i <= max; i = i + 2) {18 obj.printEven(i);19 }20 }, "T2");2122 t1.start();23 t2.start();24 }2526 public void printOdd(int number) {27 try {28 oddSemaphore.acquire();29 } catch (InterruptedException e) {30 e.printStackTrace();31 }32 System.out.println(Thread.currentThread().getName() + " : " + number);33 evenSemaphore.release();34 }3536 public void printEven(int number) {37 try {38 evenSemaphore.acquire();39 } catch (InterruptedException e) {40 e.printStackTrace();41 }42 System.out.println(Thread.currentThread().getName() + " : " + number);43 oddSemaphore.release();44 }45}