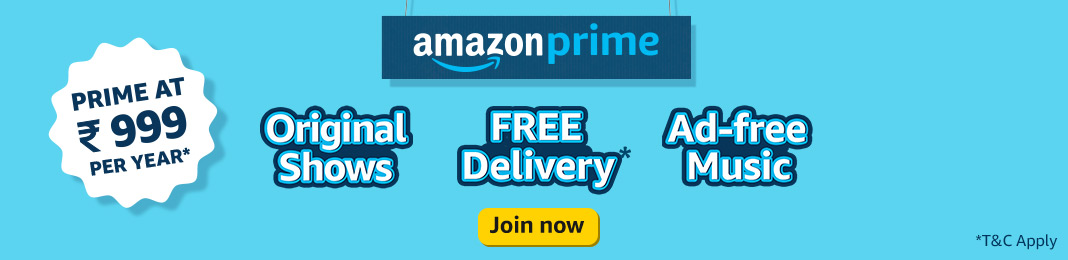
Java Program to Check Given Number is Armstrong or not
In this tutorial, we will learn how to check whether a given number is Armstrong or not.
What is Armstrong Number ?
Armstrong Number is a number that is equal to the sum of cubes of its digits. For example, 153 is an Armstrong number since 1³ + 5³ + 3³ = 153.
Algorithm
1Input : number n2Step 1: Start3Step 2: Read number n4Step 3: Initialize variable sum to 0 and temp to n5Step 4: If temp not equal to 0 Go to Step 5 , else Go to Step 96Step 5: digit = temp % 107Step 6: temp = temp / 108Step 7: sum = sum + digit * digit * digit9Step 8: Repeat Step 410Step 9: If sum is equal to n Print "Armstrong"11Step 10: Else Print "Not Armstrong"12Step 11: Stop13Output: Armstrong Number / not an Armstrong Number
Code Implementation
1import java.util.Scanner;23public class ArmstrongNumber {45 public static void main(String[] args) {6 Scanner sc = new Scanner(System.in);7 System.out.println("Enter Number : ");8 int n = sc.nextInt();9 int temp = n;10 int sum = 0;1112 while(temp !=0) {13 int digit = temp % 10;14 temp = temp / 10;15 sum = sum + digit * digit * digit ;16 }1718 if (sum == n) {19 System.out.printf("%d is an Armstrong Number \n", n);20 }else {21 System.out.printf("%d is Not an Armstrong Number \n", n);22 }23 }24}
Output 1:
1Enter Number : 1532153 is an Armstrong Number
Output 2:
1Enter Number : 3212321 is not an Armstrong Number