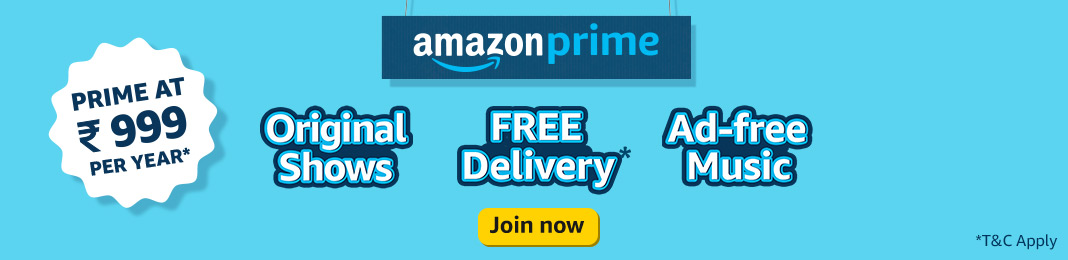
15 Posts tagged with ALGORITHM
Data Structures & Algorithms"
A data structure is a way of storing and organizing the data and an algorithm is a step by step procedure to to solve a particular problem.
Time Complexity Analysis
Time Complexity is a notation/ analysis that is used to determine how the number of steps in an algorithm increase with the increase in input size.
Introduction to Algorithms
Algorithm is a step by step procedure to solve a problem. Every algorithm takes set of inputs and compute output by following set of instructions.
Searching Algorithms
Searching Algorithms are used to determine whether given data exists within a data structure or not.
Linear Search Algorithm - Searching Algorithms
In Linear Search, we traverse the array sequentially to and check if the element is matching with the given target element or not.
Binary Search Algorithm
In Binary Search, we compare the target element with the middle element of the given sorted array and based on the outcome, we further divide the array into half and continue until we find the given element
Sorting Algorithms
Sorting algorithm is used to arrange elements of a data structure in specific order.
Bubble Sort Algorithm
Bubble Sort is the easiest sorting algorithm in which adjacent elements are compared with each other and swapped if the elements are not in proper order.
Selection Sort
Selection Sort Algorithm works by finding the minimum element of the array and placing the min element at its correct position
Divide and Conquer Approach
A divide-and-conquer algorithm recursively breaks down a problem into two or more sub problems of the same or related type, until these become simple enough to be solved directly
Recursion Algorithm
Recursion is a way of solving the given problem via solving the smaller versions of the same problem.
Two Pointer Technique
Two Pointers technique is mainly used to search for data in a given data structure. This optimization technique helps to solve the problem efficiently and effectively in terms of both time and space complexities.
Data Structures & Algorithms Problems
Min and Max of an array